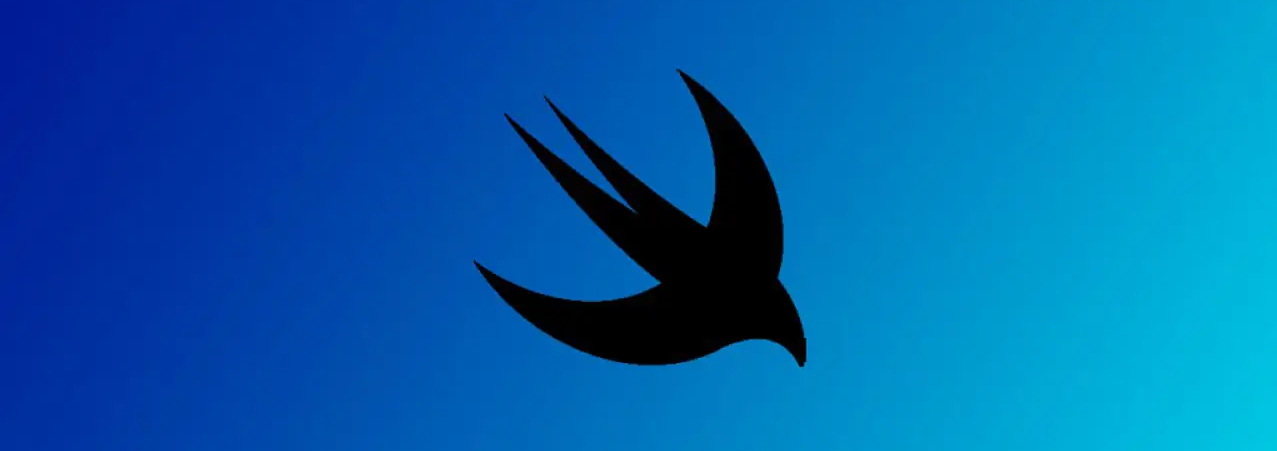
SwiftUI学习(12)-Combine入门Part VI-Applying Sequence Operations
コニクマル撰写
本文使用目前最新版本的 xcode 13 + macOS Monterey + iOS15
序列操作(Applying Sequence Operations)
drop(untilOutputFrom:)
会一直舍弃Input
发出的事件,直到参数传入的将另外一个Publisher
发出事件后才会把Input
发布的事件再发布出去。
流程图如下:
举个例子:
var store = Set<AnyCancellable>()
let input = PassthroughSubject<Int, Never>()
let publisher = PassthroughSubject<String, Never>()
let output = PassthroughSubject<Int, Never>()
input.drop(untilOutputFrom: publisher).subscribe(output).store(in: &store)
output.sink(receiveCompletion: { print("Completed ", $0)}, receiveValue: { print("Received Value ", $0)})
.store(in: &store)
input.send(1)
input.send(2)
publisher.send("any")
input.send(3)
input.send(4)
输出:
Received Value 3
Received Value 4
dropFirst(_:)
传入一个Int参数n,表示舍弃Input
发布的前n个事件,直到第n个事件后再发布出去。
流程图如下:
drop(while:)/tryDrop(while:)
传入一个闭包, 当闭包返回false的之前会把所有的Input
的事件全部丢弃,闭包返回false后,就继续发布出去。当返回false之后,这个闭包也不再回别调用了。tryDrop
可以在闭包里throw异常。
流程图如下:
append(_:)
append有三个重载版本:
-
1.传入一个动态参数列表比如
append(100, 200,300)
-
2.传入一个数组比如
append([100, 200,300])
这2个版本差不多只是参数形式不同, 当Input
发布完成事件后将,将append
内容插入到finish
之前发布出去。
流程图如下:
-
3.传入一个
Publisher
当
Input
发布完成事件后将,将Publisher
发布内容继续发布出去直到``Publisher发布
finish之后再发布
finish事件。在
Input发布的
finish之前的
Publisher`发布的事件会被丢弃:流程图如下:
举个例子:
var store = Set<AnyCancellable>() let input = PassthroughSubject<Int, Never>() let output = PassthroughSubject<Int, Never>() let publisher = PassthroughSubject<Int, Never>() input.append(publisher).subscribe(output).store(in: &store) output.sink(receiveCompletion: { print("Completed ", $0)}, receiveValue: { print("Received Value ", $0)}) .store(in: &store) input.send(1) input.send(2) input.send(3) input.send(4) publisher.send(100) input.send(completion: .finished) publisher.send(200) publisher.send(300) publisher.send(completion: .finished)
输出:
Received Value 1 Received Value 2 Received Value 3 Received Value 4 Received Value 200 Received Value 300 Completed finished
prepend(_:)
和append(_:)
正好相反,Input
之前插入事件.
prepend有三个重载版本:
-
1.传入一个动态参数列表比如
prepend(100, 200,300)
-
2.传入一个数组比如
prepend([100, 200,300])
这2个版本差不多只是参数形式不同, 马上将prepend
内容插入到第一个事件之前发布出去,无论Input
有没有发布事件。
流程图:
-
3.传入一个
Publisher
, 先发布Publisher
的事件,当Publisher
发布finish
后,才会发布Input
的事件,在此之前Input
发布的事件会被舍弃。流程图如下:
prefix(_:)
这个和dropFirst
相反,传入一个Int参数n, 只会重新发布Input
的前n个事件。之后的全部舍弃。当发布完第n元素后发布finish
流程图如下:
prefix(while:)/tryPrefix(while:)
和drop(while:)/tryDrop(while:)
相反,传入一个闭包, 当闭包返回false的之前会把所有的Input
的事件重新发布出去,闭包返回false发布finish
。tryPrefix
可以在闭包里throw异常。
流程图:
prefix(untilOutputFrom:)
和drop(untilOutputFrom:)
相反, 传入一个参数Publisher
,重新发布Input
发布的事件,直到Publisher
发布第一个事件后,发布finish
。
流程如下: